C Technology: A Comprehensive Guide
C Technology, a cornerstone of modern computing, has profoundly shaped the landscape of software development. From operating systems to embedded systems, C’s versatility and efficiency have made it a language […]
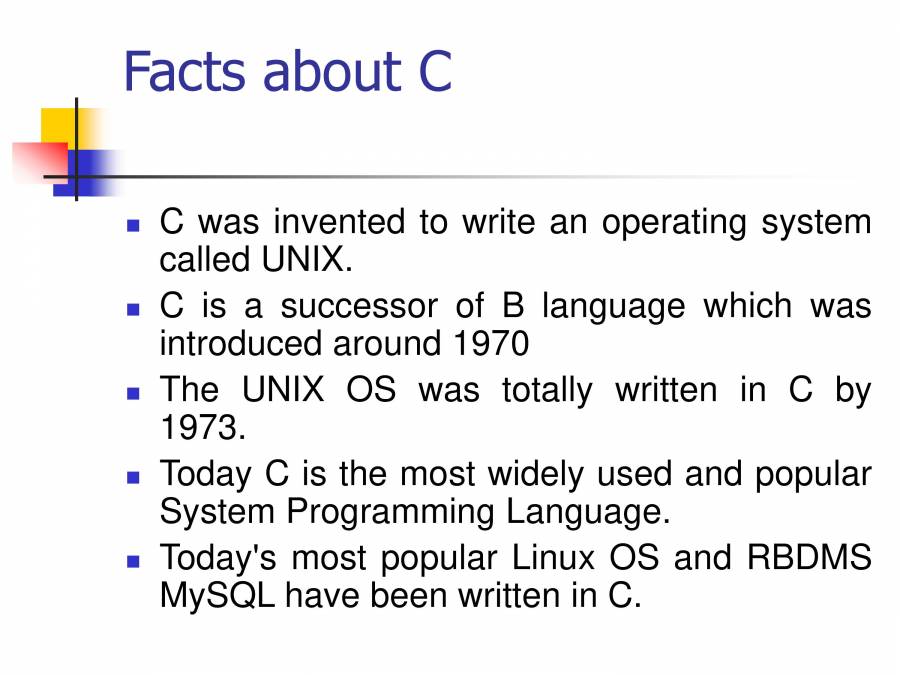
C Technology, a cornerstone of modern computing, has profoundly shaped the landscape of software development. From operating systems to embedded systems, C’s versatility and efficiency have made it a language of choice for countless applications. This comprehensive guide delves into the intricacies of C, exploring its fundamentals, programming concepts, and real-world applications.
This exploration begins with a deep dive into the origins and evolution of C, tracing its path from its humble beginnings to its current status as a widely adopted language. We’ll examine the core syntax and grammar, providing practical examples to solidify understanding. A thorough analysis of pointers, their role in memory management, and their impact on program efficiency will be included.
C Language Fundamentals
The C programming language is a powerful and versatile tool used for developing a wide range of applications, from operating systems to embedded systems. Its history and evolution have shaped its features and influence on modern programming.
History and Evolution of C
The C programming language was developed by Dennis Ritchie at Bell Labs in the early 1970s. It was initially designed to be a system programming language for the Unix operating system, but its flexibility and efficiency quickly made it popular for other purposes. C evolved through several iterations, including the standardization by the American National Standards Institute (ANSI) in 1989 and the subsequent adoption of the ISO standard in 1990. These standards ensured consistency and portability across different platforms.
Core Syntax and Grammar Rules, C technology
C’s syntax is concise and structured. Here are some key elements:
* s: C uses reserved words with specific meanings, such as `int`, `float`, `for`, and `while`.
* Identifiers: These are names used to identify variables, functions, and other program elements.
* Data Types: C supports various data types to represent different kinds of values, including integers, floating-point numbers, characters, and pointers.
* Operators: These symbols perform operations on data, such as arithmetic, relational, and logical operators.
* Expressions: Combinations of operators, variables, and constants that evaluate to a value.
* Statements: Instructions that perform actions, such as assignment, function calls, and control flow statements.
* Comments: Used to explain the code and improve readability.
Basic C Programs
Here are some examples of basic C programs that demonstrate fundamental concepts:
1. Hello World:
“`c
#include
int main()
printf(“Hello, World!\n”);
return 0;
“`
This program prints the message “Hello, World!” to the console. It uses the `printf()` function from the `stdio.h` header file.
2. Adding Two Numbers:
“`c
#include
int main()
int num1, num2, sum;
printf(“Enter two numbers: “);
scanf(“%d %d”, &num1, &num2);
sum = num1 + num2;
printf(“Sum: %d\n”, sum);
return 0;
“`
This program takes two numbers as input from the user, adds them together, and displays the result. It demonstrates the use of variables, input/output operations, and arithmetic operators.
3. Using Control Flow Statements:
“`c
#include
int main()
int num;
printf(“Enter a number: “);
scanf(“%d”, &num);
if (num > 0)
printf(“The number is positive.\n”);
else if (num < 0)
printf("The number is negative.\n");
else
printf("The number is zero.\n");
return 0;
“`
This program determines whether a number is positive, negative, or zero using `if-else` statements. It illustrates conditional execution based on a condition.
Data Types in C
Data types define the kind of data that a variable can hold. Here is a table summarizing the common data types in C:
Data Type | Size (Bytes) | Typical Uses |
---|---|---|
`char` | 1 | Storing single characters, like letters, digits, and symbols. |
`int` | 4 | Storing whole numbers, both positive and negative. |
`float` | 4 | Storing single-precision floating-point numbers, which can represent numbers with decimal points. |
`double` | 8 | Storing double-precision floating-point numbers, which offer greater precision than `float`. |
`void` | N/A | Indicates the absence of a return value or a function that doesn’t return anything. |
Pointers in C
Pointers are variables that store memory addresses. They are crucial for:
* Direct Memory Access: Pointers allow you to access and manipulate data directly in memory.
* Dynamic Memory Allocation: Pointers are used with functions like `malloc()` and `free()` to allocate and deallocate memory during program execution.
* Passing Data to Functions: Pointers enable efficient data sharing between functions by passing memory addresses instead of copying entire data structures.
* Creating Linked Lists and Other Data Structures: Pointers are essential for building dynamic data structures that can grow or shrink in size as needed.
Example:
“`c
int *ptr; // Declaring a pointer to an integer
int num = 10;
ptr = # // Assigning the address of ‘num’ to ‘ptr’
printf(“%d\n”, *ptr); // Dereferencing the pointer to access the value at the address it points to
“`C Standard Library: C Technology
The C standard library is a collection of pre-written functions and macros that provide a wide range of functionalities, simplifying the development process and reducing the amount of code you need to write from scratch. These functions are available in every C compiler and provide a consistent way to perform common tasks.Key Components
The C standard library is organized into different headers, each providing a specific set of functions. Some of the most important headers include:
- stdio.h: Provides input/output functions like
printf
,scanf
,fopen
, andfclose
.- stdlib.h: Offers general-purpose functions, including memory allocation (
malloc
,free
), string conversion (atoi
,atof
), random number generation (rand
), and process control (exit
,system
).- string.h: Contains functions for manipulating strings, such as
strcpy
,strcat
,strlen
, andstrcmp
.- math.h: Provides mathematical functions, including trigonometric functions (
sin
,cos
,tan
), logarithmic functions (log
,log10
), and power functions (pow
,sqrt
).- time.h: Offers functions for working with time and date, such as
time
,ctime
, anddifftime
.- ctype.h: Provides functions for character classification and conversion, such as
isalpha
,isdigit
,toupper
, andtolower
.Using Standard Library Functions
String Manipulation
strcpy(destination, source)
: Copies a string fromsource
todestination
.strcat(destination, source)
: Concatenates a string fromsource
to the end ofdestination
.strlen(string)
: Returns the length of a string.strcmp(string1, string2)
: Compares two strings lexicographically and returns 0 if they are equal, a negative value ifstring1
is less thanstring2
, and a positive value ifstring1
is greater thanstring2
.Example:
“`c
#include
#includeint main()
char str1[50] = “Hello “;
char str2[] = “World!”;strcpy(str1, “Welcome “); // Overwrites str1
strcat(str1, str2); // Concatenates str2 to str1printf(“Concatenated string: %s\n”, str1);
printf(“Length of string: %zu\n”, strlen(str1));return 0;
“`
Mathematical Operations
sqrt(x)
: Calculates the square root ofx
.pow(x, y)
: Calculatesx
raised to the power ofy
.sin(x)
,cos(x)
,tan(x)
: Calculates the sine, cosine, and tangent ofx
, respectively.Example:
“`c
#include
#includeint main()
double x = 25.0;
double y = 3.0;printf(“Square root of %lf: %lf\n”, x, sqrt(x));
printf(“%lf raised to the power of %lf: %lf\n”, x, y, pow(x, y));return 0;
“`
File Handling
fopen(filename, mode)
: Opens a file with the specifiedfilename
andmode
(e.g., “r” for reading, “w” for writing, “a” for appending).fclose(file)
: Closes a file previously opened withfopen
.fprintf(file, format, ...)
: Writes formatted output to a file.fscanf(file, format, ...)
: Reads formatted input from a file.Example:
“`c
#includeint main()
FILE *file = fopen(“output.txt”, “w”); // Open file for writingif (file != NULL)
fprintf(file, “This is a line of text written to a file.\n”);
fclose(file); // Close the file
printf(“File written successfully!\n”);
else
printf(“Error opening file!\n”);return 0;
“`
Advantages and Disadvantages
- Advantages:
- Efficiency: Standard library functions are highly optimized for performance, often utilizing assembly language or hardware-specific instructions.
- Portability: The C standard library is designed to be portable across different operating systems and architectures, ensuring your code can run on a variety of platforms without significant changes.
- Consistency: Using standard library functions provides a consistent and reliable way to perform common tasks, promoting code readability and maintainability.
- Disadvantages:
- Limited Flexibility: Standard library functions may not always offer the exact functionality or customization required for specific tasks. You may need to write your own functions in these cases.
- Potential Overhead: While optimized, using standard library functions can introduce some overhead compared to hand-coded solutions, especially in performance-critical applications.
Commonly Used Functions
Header Function Purpose Parameters stdio.h printf
Prints formatted output to the console. Format string and optional arguments. stdio.h scanf
Reads formatted input from the console. Format string and pointers to variables. stdlib.h malloc
Allocates a block of memory. Size of the memory block in bytes. stdlib.h free
Frees a block of memory previously allocated with malloc
.Pointer to the memory block. string.h strcpy
Copies a string from one location to another. Destination string and source string. string.h strcat
Concatenates two strings. Destination string and source string. string.h strlen
Returns the length of a string. String. math.h sqrt
Calculates the square root of a number. Number. math.h pow
Raises a number to a power. Base number and exponent. time.h time
Gets the current time. Pointer to a time_t
variable.Last Point
As we conclude our journey through the world of C Technology, we’ve witnessed its remarkable power and enduring relevance. From its foundational principles to its diverse applications, C continues to be a vital tool for programmers of all levels. Its ability to interact with hardware, optimize performance, and provide a foundation for higher-level languages ensures its continued significance in the ever-evolving landscape of software development.
C technology is a cornerstone of software development, providing a foundation for countless applications. From operating systems to embedded systems, its efficiency and control are highly valued. For companies seeking to implement robust and scalable solutions, partnering with a trusted technology provider like bradley technologies can be a strategic advantage.
Their expertise in C technology can help streamline development processes and ensure high-quality results. Ultimately, the success of any project relies on a strong foundation, and C technology, when leveraged effectively, can provide just that.